核心概念:Subject, SecurityManager, and Realms
Subject
####Subject is a security term that basically means "the currently executing user"
#####Acquiring the Subject
import org.apache.shiro.subject.Subject;
import org.apache.shiro.SecurityUtils;
……
Subject currentUser = SecurityUtils.getSubject();
#####如果拿到了subject,shiro90%的事情都可以做了例如
- login
- logout
- access their session
- execute authorization checks
SecurityManager
The SecurityManager manages security operations for all users.
How do we set up a SecurityManager?
如果是web应用,我们通常会在web.xml指定一个Shiro Servlet Filter,它会创建一个SecurityManager 实例。SecurityManager 一般是一个单例,他的默认实现是POJO,配置形式有如下几种方式:
- normal Java code
- Spring XML
- YAML
- .properties
- .ini files
ini files是最常用的,因为 INI is easy to read, simple to use, and requires very few dependencies。for example
1.用ini配置shiro
[main]
cm = org.apache.shiro.authc.credential.HashedCredentialsMatcher
cm.hashAlgorithm = SHA-512
cm.hashIterations = 1024
# Base64 encoding (less text):
cm.storedCredentialsHexEncoded = false
iniRealm.credentialsMatcher = $cm
[users]
jdoe = TWFuIGlzIGRpc3Rpbmd1aXNoZWQsIG5vdCBvbmx5IGJpcyByZWFzb2
asmith = IHNpbmd1bGFyIHBhc3Npb24gZnJvbS文件ciBhbXNoZWG5vdCB
INI File解析
1). main区域是用来配置SecurityManager 对象的,这里设置了两个对象,crm以及iniRealm。并且m对象设置了一些参数。然后将crm赋值给了iniRealm对象。
2). users区域用来指定静态的用户账号。
2.java类加载ini配置文件
import org.apache.shiro.SecurityUtils;
import org.apache.shiro.config.IniSecurityManagerFactory;
import org.apache.shiro.mgt.SecurityManager;
import org.apache.shiro.util.Factory;
//1. Load the INI configuration
Factory<SecurityManager> factory =
new IniSecurityManagerFactory("classpath:shiro.ini");
//2. Create the SecurityManager
SecurityManager securityManager = factory.getInstance();
//3. Make it accessible
SecurityUtils.setSecurityManager(securityManager);
代码解析
1)加载ini配置文件
2)创建SecurityManager实例
3)让SecurityManager实例可以被应用访问
Realms
#### A Realm acts as the ‘bridge’ or ‘connector’ between Shiro and your application’s security data
######做用户账号(security-related data)和登录(authentication)以及访问控制(authorization )之间的交互
#####Shiro 提供多种开箱即用的安全数据源:
LDAPelational databases :JDBCtext configuration sources : INI , properties files,and more
Authentication
1. 获取用户的登录名 (principals),和密码(credentials).
2. 将上一步获取的信息提交给系统
3. 如果跟系统期待的匹配,用户是已认证的,否则是未认证的
用户登录
//1. Acquire submitted principals and credentials:
AuthenticationToken token =
new UsernamePasswordToken(username, password);
//2. Get the current Subject:
Subject currentUser = SecurityUtils.getSubject();
//3. Login:
currentUser.login(token);
处理失败的情况
//3. Login:
try {
currentUser.login(token);
} catch (IncorrectCredentialsException ice) { …
} catch (LockedAccountException lae) { …
}
…
catch (AuthenticationException ae) {…
}
Authorization
Authorization is essentially access control - controlling what your users can access in your application
Authorization是用来控制用户可以访问应用的哪些resource和webpage
Subject API 让我们以非常简便的方式做role和permission校验.Subject Api Permission Document例如:
if ( subject.hasRole(“administrator”) ) {
//show the ‘Create User’ button
} else {
//grey-out the button?
}
……
if ( subject.isPermitted(“user:create”) ) {
//show the ‘Create User’ button
} else {
//grey-out the button?
}
……
if ( subject.isPermitted(“user:delete:jsmith”) ) {
//delete the ‘jsmith’ user
} else {
//don’t delete ‘jsmith’
}
Session Management
Shiro enables a Session programming paradigm for any application - from small daemon standalone applications to the largest clustered web applications.
Shiro’s architecture allows for pluggable Session data stores,And it is container independent.
实例
Session session = subject.getSession();
Session session = subject.getSession(boolean create);
session.getAttribute(“key”, someValue);
Date start = session.getStartTimestamp();
Date timestamp = session.getLastAccessTime();
session.setTimeout(millis);
Cryptography
Web Support
Shiro ships with a robust web support module to help secure web applications.ReferShiro Web
ShiroFilter in web.xml
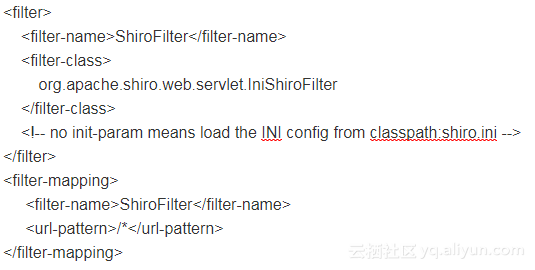
####URL-Specific Filter Chains
#####Shiro supports security-specific filter rules through its innovative URL filter chaining capability
######Shiro支持一种创造性的URL安全过滤器,例如
[urls]
/assets/** = anon
/user/signup = anon
/user/** = user
/rpc/rest/** = perms[rpc:invoke], authc
/** = authc
左边是URL是web应用的相对路径,右边是过滤器链
JSP Tag Library
Web Session Management
1.Default Http Sessions
Shiro defaults its session infrastructure to use the existing Servlet Container sessions that we’re all used to.
2.Shiro’s Native Sessions in the Web Tier